What impact does JavaScript have on the speed and performance of a website?
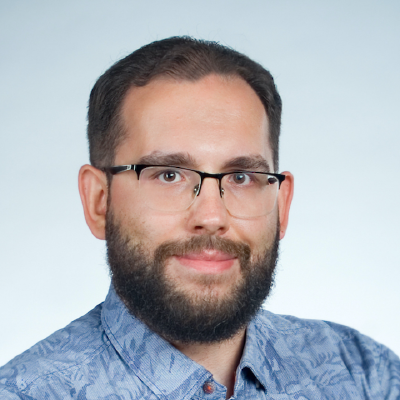
Of all the elements that go into making a website, scripts can be the most versatile. However, going hand-in-hand with their versatility is the potential for scripts to have a huge impact on website performance, making them a key part of any optimisation strategy. They are also often a weak point that hackers can use to attack a site, which is why it's important that we regularly analyse and optimise our scripts regularly to ensure our sites remain both safe and fast.
In this article we'll go over some key areas to keep in mind when looking to optimise our scripts, and highlight some best practices that will help our website stay safe from unwanted intrusions.
Stay up-to-date with JavaScript API development
Often, when implementing a new feature on their websites, users will utilise pre-built code snippets designed by other developers rather than writing the code themselves. This has a dual benefit; inexperienced developers are able to take advantage of more advanced features that would be difficult for them to code alone, and even experienced users can appreciate the time saved by using a pre-written piece of code.
However, as browsers are updated and improved they will often add support for features that previously were only possible through scripts. Failing to adapt our content to these innovations can prevent our sites from reaching their full potential, so its important to keep abreast of new features and functions that are introduced in browser updates to keep our sites running smoothly and efficiently. Here are three examples of issues that relatively-new browser functions can solve much more efficiently:
Example 1: lazy load of images
Up until a few years ago, if we wanted to lazy-load images (that is, load images only when the user scrolls to the image instead of on the initial page load, letting users see page content faster), a very common solution was to replace the src attribute in images with the data-src attribute and then tracking a user's scrolling through the site via the onscroll event of the browser window.
Today, fortunately, this approach is slowly becoming a relic of the past due to the introduction of loading attribute , which allows us to perform the same function without running additional scripts.
Example 2: Activating items while scrolling the page
Over the years we've gotten a lot of mileage out of the onscroll event; as well as using it for lazy-loading images, it was also often used to trigger events at certain points of a given webpage. For example, we might want a sticky menu to appear once the user scrolls away from the top of the page. Currently, a more robust solution is the IntersectionObserver API; with this, we can trigger events at a specific moment relative to the visibility of a page element or group of elements. This makes it an ideal solution for sticky menus, animations that trigger during scrolling, or simply for analytics, tracking whether a given item has been viewed by the user.
Example 3: tracking website changes
It can be quite a challenge to link our website scripts with other external scripts; if our scripts don't utilise a convenient API or cause events of any kind, then we don't have a way to monitor changes. This can be overcome through cyclically executing scripts that will verify whether there has been any change important to our scripts on the page, but it is much better to use the MutationObserver API, which allows us to track changes taking place in specific elements at different levels - whether HTML structure, text or attributes.
This provides a number of opportunities for both optimizing our scripts and integrating them with external scripts.
Compress and minify scripts
As with CSS and HTML, it's always a good idea to minify and compress your JavaScript code to save bandwidth and reduce the number of queries that your site needs to run when loading.
In the case of JS code, tools to minify scripts have much more scope than that of CSS code, because there are often much easier ways to simplify the script construction, though this fo course makes them less readable should you wish to examine the scripts later.
In addition, we should pay careful attention to the order that our scripts are loaded; an inefficient load order can lead to unnecessary load delays for the user and an overall worse user experience.
Avoid inline
As a general rule, we should avoid embedding scripts into our HTML code, especially if they have references to other libraries, as in order to use these libraries they must be loaded in the <head>
section of our website, which will, in turn, cause significant delays in page-rendering time.
If you find yourself in a situation where you absolutely must rely on inline JS code, try to use simple, vanilla JS code without any additional dependencies to mitigate the number of references needed.
Place scripts at the end of a document
When preparing scripts, we should assume that they will be loaded in the footer of the page; this makes it easier to write them as we can be sure that all of our required HTML elements will be loaded, ready to be modified before the scripts are executed; no need to perform any checks.
The only scripts that should be included in the <head>
section or in the page code, are the most important ones that need to be executed immediately (e.g. code from a cookie banner or a script telling the browser that JS is available by removing the no-js class from the HTML tag).
Thanks to this, the page will render much faster because it will not be delayed by loading scripts.
Give up jQuery
jQuery is getting on a bit (to put it mildly), but it can still make your life easier. However, browser APIs are not static, and we can generally achieve the same results with just a little bit more code as we could with jQuery. Rather than tying ourselves to an older library, we should ensure our website is prepared for the modern era.
It is worth checking this website for a wealth of information on alternative approaches to common jQuery solutions: https://youmightnotneedjquery.com/
By foregoing jQuery on our website, we can get rid of several dozen kilobytes of code, a large part of which is never used.
Watch out for dependencies
When choosing scripts to be used on the website, pay attention to their possible applications beyond what you are currently using them for; the more universal the solution, the greater the chance that the script will be larger than necessary and will negatively affect the size of the page.
Often scripts will load their dependencies separately (sometimes from external servers), so it's a good idea to check out what’s happening in the "Network" tab of development tools once the new solution has been implemented on your site to see if there are any blips where new dependencies are being loaded.
Support JS with CSS code
The amount of JS code can be reduced in one more way - by using CSS code. The idea is to implement as many traditionally script-based parts of your site using pure CSS code instead, at least partially.
For example, when making a photo slider, instead of writing code that animates all the slider elements, you can create a script that will update a specific attribute, e.g. data-current-slide, and create all the animation logic in CSS dependent on this attribute:
.items[data-current-slide="1"] {
transform: translateX(0%);
}
.items[data-current-slide="2"] {
transform: translateX(-25%);
}
.items[data-current-slide="3"] {
transform: translateX(-50%);
}
.items[data-current-slide="4"] {
transform: translateX(-75%);
}
Additionally, using a CSS preprocessor, eg SCSS, you can generate such rules quite quickly in advance (although make sure to apply a bit of common sense here; we don't want to grow our CSS code so much as to render the optimizations obsolete).
Be careful with analytics!
Analytics scripts offer great opportunities for collecting and analyzing data that can be used to optimize both our site and user experience. However, if we put several different tracking codes in our website, including a chat and a HotJar script, our website has basically no chance of getting good results in Page Speed tests, because the analytics scripts themselves will significantly slow down our website.
I'm not suggesting that you should completely abandon analytics; after all, the data we receive can offer benefits to both you and your users. However, we should focus our analytics on one or two services and use their full potential for data analysis, instead of thoughtlessly sending user data to a dozen places without even having time to analyze the data collected.
Load complex scripts when you really need them
No matter what we do, there are going to be some cases where a heavy script must be loaded to provide the services we want to offer on our site. But even here we may find options to optimize and streamline the user experience to avoid unnecessary delays. Rather than loading all your heavy scripts right on the page, consider if there is a way to wait for a user prompt before loading the script. For example:
- Do you have chat on the site?
Display a chat icon that, when clicked, will load the chat script. - Do you need a captcha solution to block bots in the contact form?
Load the scripts only after the user starts filling out the form. - Do you want to show the user how to get to you on the map?
Instead of a map, add a "See how to find us" button on the website with a link to the map, or open a new popup with the map once the button is clicked. - Do you want to allow users to view an interactive directory?
Display an image with a preview of the directory, which when clicked will load the directory viewer script.
Summarize
The use of JavaScript on a website requires moderation and a common-sense approach to balance its functionality with our actual needs. It’s very easy to get carried away and overload the page with loads of unnecessary effects and features. Take the time to consider exactly what you need your site to do, and regularly verify the scripts used, and consider whether there are alternative, better solutions for adding these features.